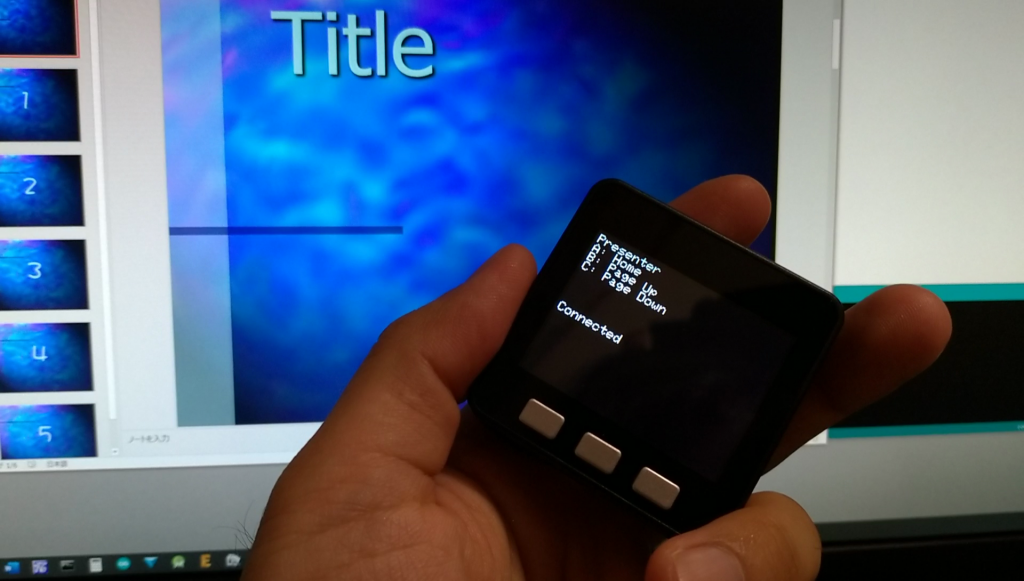
ESP32 devices can be used as Bluetooth device, such as mouse or keyboard. It was hard to write sketch while ago, but now, there’s handy library which you can easily make ESP32 to Bluetooth hid device.
This time, I’ll be using M5Stack to work as simple PowerPoint controller.
Download and install library from URL below for Bluetooth Keyboard.
https://github.com/T-vK/ESP32-BLE-Keyboard
After installing library is simple. Call begin, then press/release function to send key. Since M5Stack have 3 buttons, I’ve assigned Home, Page Up, and Page Down key to them. You can also do some more complicated control using long hold button, etc.
#include <M5Stack.h>
#include <BleKeyboard.h>
BleKeyboard bleKeyboard("M5 Presenter");
bool blestate = false;
void showstate(char *txt) {
M5.Lcd.setCursor(0, 120);
M5.Lcd.fillRect(0, 120, 320, 20, BLACK);
M5.Lcd.printf(txt);
}
void setup() {
M5.begin();
M5.Power.begin();
bleKeyboard.begin();
M5.Lcd.clear(BLACK);
M5.Lcd.setTextSize(2);
M5.Lcd.println("Presenter");
M5.Lcd.println("A: Home");
M5.Lcd.println("B: Page Up");
M5.Lcd.println("C: Page Down");
showstate("Disconnected");
}
void loop() {
M5.update();
if (bleKeyboard.isConnected()) {
if (!blestate) {
blestate = true;
showstate("Connected");
}
if (M5.BtnA.wasPressed()) {
bleKeyboard.press(KEY_HOME);
bleKeyboard.release(KEY_HOME);
} else if (M5.BtnB.wasPressed()) {
bleKeyboard.press(KEY_PAGE_UP);
bleKeyboard.release(KEY_PAGE_UP);
} else if (M5.BtnC.wasPressed()) {
bleKeyboard.press(KEY_PAGE_DOWN);
bleKeyboard.release(KEY_PAGE_DOWN);
}
} else {
if (blestate) {
blestate = false;
showstate("Disconnected");
}
}
}
After writing sketch to M5Stack and booting, you will find M5Stack as Bluetooth device with name “M5 Presenter”. Pair and you are ready.
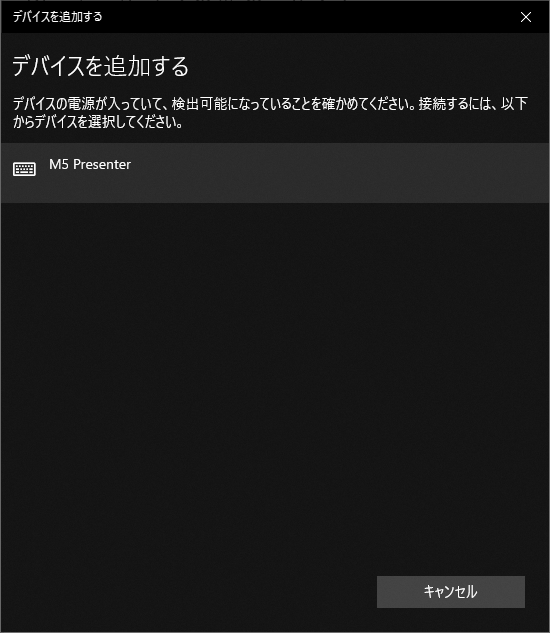
Press 3 buttons on M5Stack, and it will work as Home, Page UP, and Page Down key.
See below for quick video. Amazingly simple.
This post is also available in: Japanese