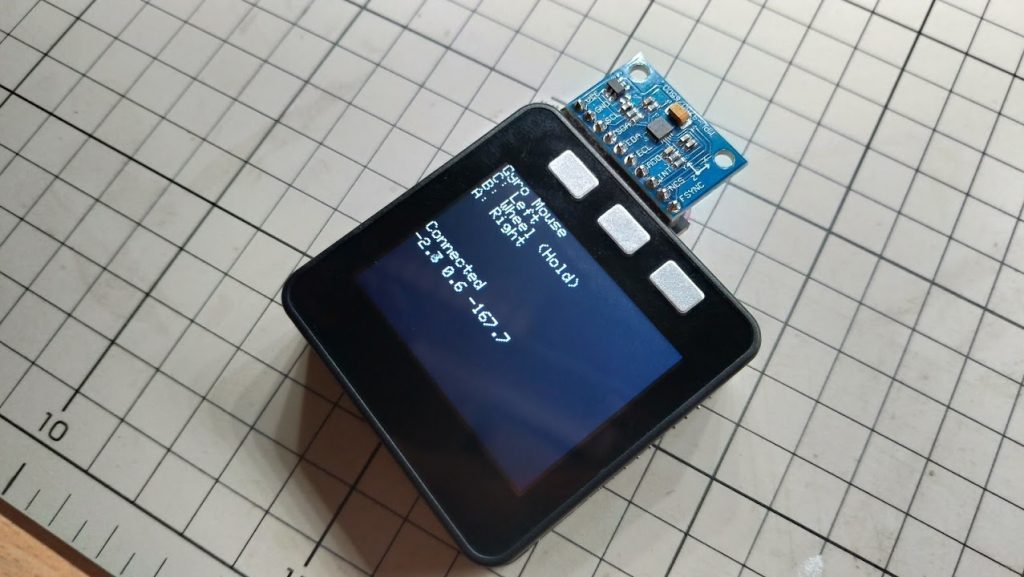
Thanks to author of library, Bluetooth mouse library is available for ESP32 devices. I’ll be making Bluetooth mouse with M5Stack this time.
https://github.com/T-vK/ESP32-BLE-Mouse
Download and install library from above site.
There are many ideas, such us using joystick, to control mouse. This time, I’ll use MPU9250 gyro sensor. Connect sensor with I2C wiring.
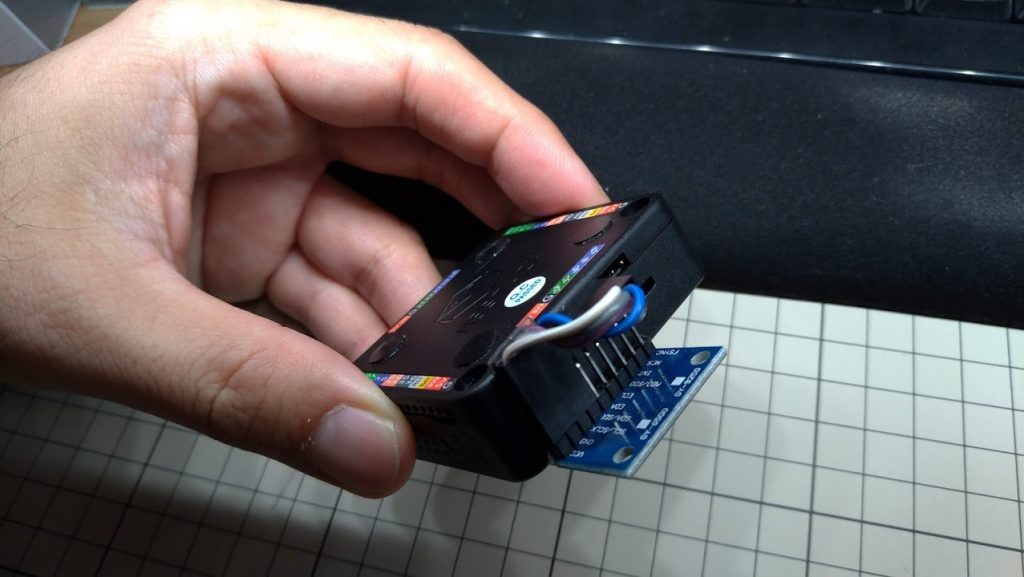
There are M5Stack models which has gyro sensor inside. For those M5Stack models, only thing you need is M5Stack itself only.
Tweet from M5Stack official below shows good table of difference between models. Check MEMS row.
Sketch is as follows. M5Stack is used upside down. Button C for left click, A for right click, hold down B and tilt for scroll.
Gyro sensor are also simplified with library; sketch is kept neat and simple.
#define M5STACK_MPU9250
#include <M5Stack.h>
#include <BleMouse.h>
#define GYRO_CHECK_INTERVAL 10
#define GYRO_CHECK_INTERVAL_WHEEL 30
BleMouse bleMouse("M5 GyroMouse");
bool blestate = false;
bool wheelmode = false;
int gyrointerval = GYRO_CHECK_INTERVAL;
signed char mousex, mousey, mwheel;
int ipitch, iroll, iyaw;
float pitch, roll, yaw;
char chbuff[32];
unsigned long current_millis, last_millis = 0;
void showstate(char *txt) {
M5.Lcd.setCursor(0, 120);
M5.Lcd.fillRect(0, 120, 320, 20, BLACK);
M5.Lcd.printf(txt);
}
void showgyro(char *txt) {
M5.Lcd.setCursor(0, 140);
M5.Lcd.printf(txt);
}
void setup() {
M5.begin();
M5.Power.begin();
bleMouse.begin();
M5.Lcd.clear(BLACK);
M5.Lcd.setRotation(3);
M5.Lcd.setTextSize(2);
M5.Lcd.println("Gyro Mouse");
M5.Lcd.println("C: Left");
M5.Lcd.println("B: Wheel (Hold)");
M5.Lcd.println("A: Right");
showstate("Disconnected");
M5.IMU.Init();
}
void loop() {
M5.update();
current_millis = millis();
if (bleMouse.isConnected()) {
if (!blestate) {
blestate = true;
showstate("Connected");
}
if (current_millis - last_millis > gyrointerval) {
M5.IMU.getAhrsData(&pitch, &roll, &yaw);
sprintf(chbuff, "%.1f %.1f %.1f ", pitch, roll, yaw);
ipitch = (int)pitch;
iroll = (int)roll;
iyaw = (int)yaw;
showgyro(chbuff);
if (wheelmode) {
mousex = 0;
mousey = 0;
if (abs(ipitch) >= 10)
mwheel = (signed char)(ipitch / 10);
} else {
mousex = (signed char)((iroll < 0 ? min(0, iroll + 10) : max(0, iroll - 10)) / 10);
mousey = -1 * (signed char)((ipitch < 0 ? min(0, ipitch + 10) : max(0, ipitch - 10)) / 10);
mwheel = 0;
}
bleMouse.move(mousex, mousey, mwheel);
last_millis = current_millis;
}
if (M5.BtnA.wasPressed()) {
bleMouse.press(MOUSE_RIGHT);
} else if (M5.BtnA.wasReleased()) {
bleMouse.release(MOUSE_RIGHT);
} else if (M5.BtnB.wasPressed()) {
wheelmode = true;
gyrointerval = GYRO_CHECK_INTERVAL_WHEEL;
} else if (M5.BtnB.wasReleased()) {
wheelmode = false;
gyrointerval = GYRO_CHECK_INTERVAL;
} else if (M5.BtnC.wasPressed()) {
bleMouse.press(MOUSE_LEFT);
} else if (M5.BtnC.wasReleased()) {
bleMouse.release(MOUSE_LEFT);
}
} else {
if (blestate) {
blestate = false;
showstate("Disconnected");
}
}
}
Uploaded youtube video showing M5Stack paired with PC. Somehow, I was unable to pair with GPD MicroPC.
This post is also available in: Japanese