ESP8266 / ESP32 can be easily used as Web server. Also, ESP device can connect to internet via Wi-Fi, or itself can be used as Access Point.
Some usage are, connect to ESP device from Smartphone, open web page, configure setting or control peripheral device.
E.g. ESP as smart plug controller, configure on/off scheduling time, or ESP as remote-controlled robot with servo and motor, and control using browser interface.
One annoying thing when using ESP as access point / web server is, after connecting to ESP device from Smartphone, I have to open browser and type in IP address in URL and open page.
Well, this can be automated using Captive Portal technology.
You might have seen “Disclaimer” or “Sign in” web page after connecting to free Wi-Fi spot at airport, hotel, or stores. This is using Captive Portal.
Simple sketch below is basic code for ESP8266. When you run this sketch, ESP8266 will run as Access Point.
Connect to ESP8266 Access Point from Smartphone. It may differ depending on OS or type of Smartphone; popup notification asking you to Login shows up.
When you tap login, ESP8266 web page shows up. Quick and easy!
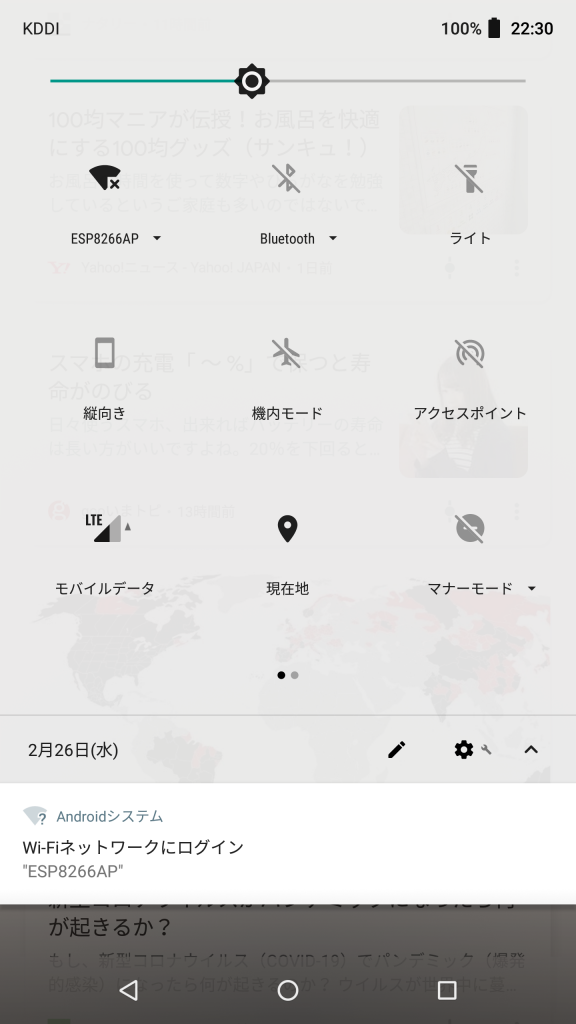
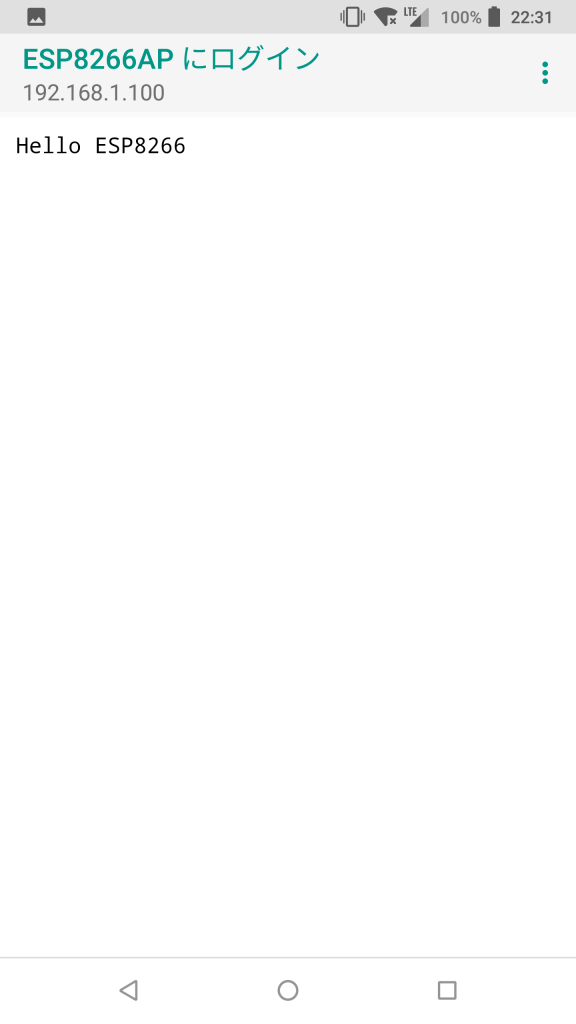
Now you don’ t have to remember ESP device IP address or hostname to access.
#include <ESP8266WiFi.h>
#include <ESP8266WebServer.h>
#include <ESP8266mDNS.h>
#include <DNSServer.h>
const char* ap_ssid = "ESP8266AP"; //AP SSID
const char* ap_password = "12345678"; //AP password 8 character or more
IPAddress ip(192, 168, 1, 100);
IPAddress subnet(255, 255, 255, 0);
const byte DNS_PORT = 53;
DNSServer dnsServer;
ESP8266WebServer server(80);
String toStringIp(IPAddress ip) {
String res = "";
for (int i = 0; i < 3; i++) {
res += String((ip >> (8 * i)) & 0xFF) + ".";
}
res += String(((ip >> 8 * 3)) & 0xFF);
return res;
}
void captivePortal() {
server.sendHeader("Location", String("http://") + toStringIp(server.client().localIP()), true);
server.send(302, "text/plain", "");
server.client().stop();
}
bool handleUrl(String path) {
if (path.endsWith("/")){
char chbuffer[64];
sprintf(chbuffer,"Hello ESP8266");
server.send(200,"text/plain",chbuffer);
return true;
}
return false;
}
void setup() {
WiFi.mode(WIFI_AP);
WiFi.softAPConfig(ip, ip, subnet);
WiFi.softAP(ap_ssid, ap_password);
dnsServer.start(DNS_PORT, "*", ip);
WiFi.setSleepMode(WIFI_NONE_SLEEP);
server.onNotFound([]() {
if (!handleUrl(server.uri())) {
captivePortal();
}
});
server.begin();
}
void loop() {
dnsServer.processNextRequest();
server.handleClient();
}
ESP32 sample.
#include <WiFi.h>
#include <WiFiClient.h>
#include <WebServer.h>
#include <ESPmDNS.h>
#include <DNSServer.h>
const char* ap_ssid = "ESP32AP"; //AP SSUD
const char* ap_password = "12345678"; //AP Password
IPAddress ip(192, 168, 1, 100);
IPAddress subnet(255, 255, 255, 0);
const byte DNS_PORT = 53;
DNSServer dnsServer;
WebServer server(80);
String toStringIp(IPAddress ip) {
String res = "";
for (int i = 0; i < 3; i++) {
res += String((ip >> (8 * i)) & 0xFF) + ".";
}
res += String(((ip >> 8 * 3)) & 0xFF);
return res;
}
void captivePortal() {
server.sendHeader("Location", String("http://") + toStringIp(server.client().localIP()), true);
server.send(302, "text/plain", "");
server.client().stop();
}
bool handleUrl(String path) {
if (path.endsWith("/")){
char chbuffer[64];
sprintf(chbuffer,"Hello ESP32");
server.send(200,"text/plain",chbuffer);
return true;
}
return false;
}
void setup() {
WiFi.mode(WIFI_AP);
WiFi.softAPConfig(ip, ip, subnet);
WiFi.softAP(ap_ssid, ap_password);
dnsServer.start(DNS_PORT, "*", ip);
server.onNotFound([]() {
if (!handleUrl(server.uri())) {
captivePortal();
}
});
server.begin();
}
void loop() {
dnsServer.processNextRequest();
server.handleClient();
}
This post is also available in: Japanese